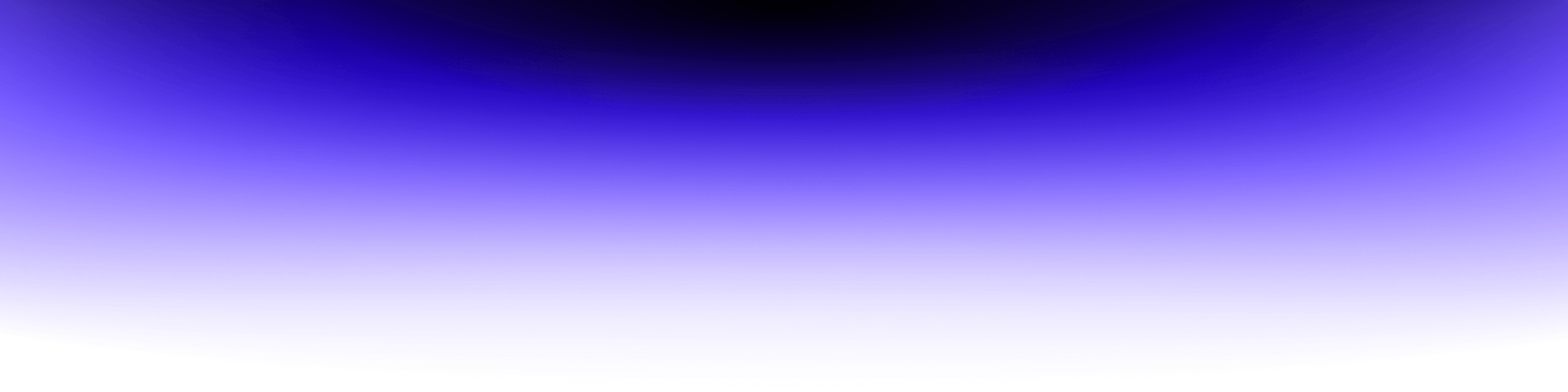
Comprehensive Strategies for Performance Enhancement in Flutter Applications
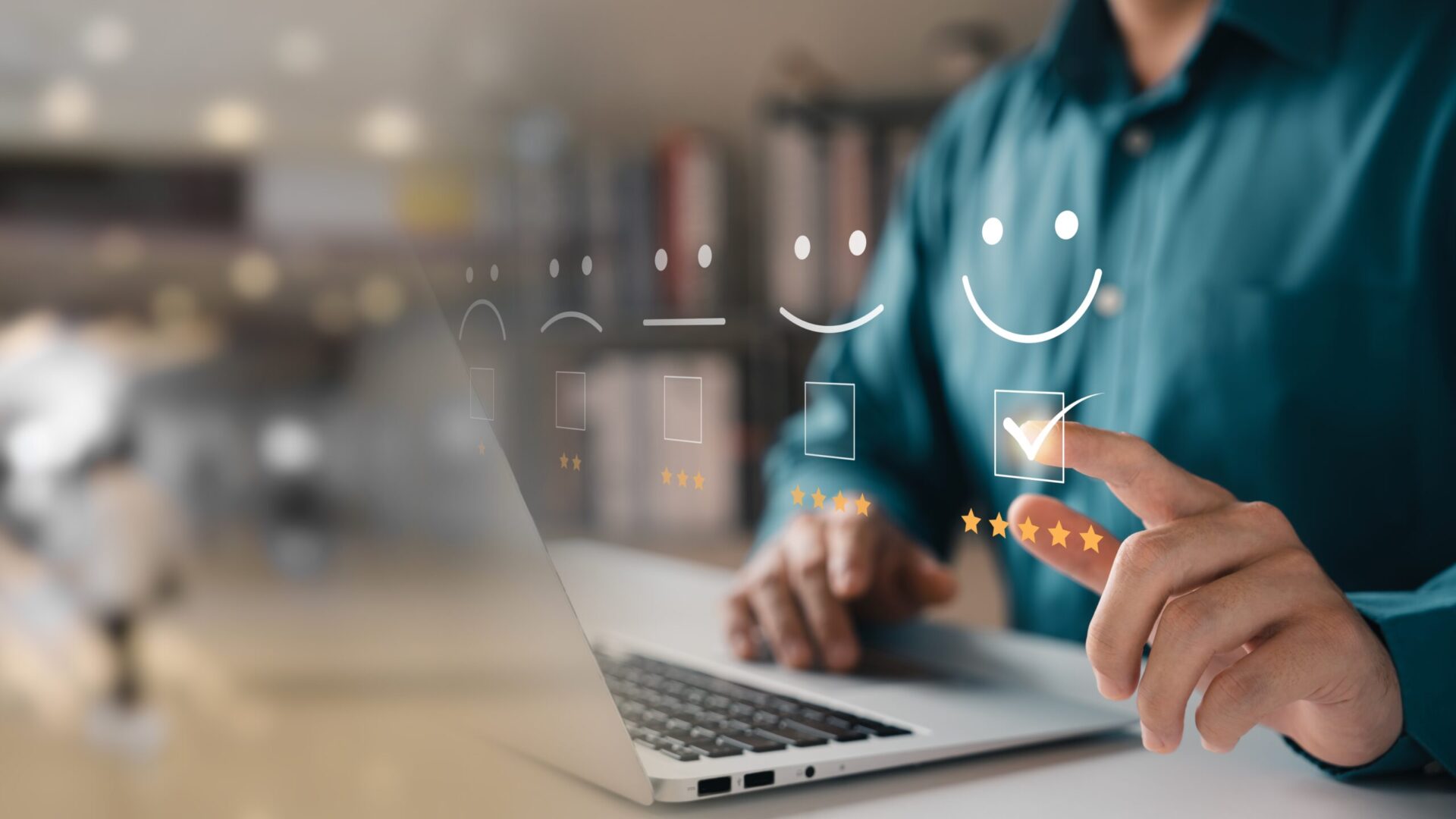
Achieving peak performance in Flutter applications is a pursuit that requires careful planning, optimization, and strategic decision-making. In this article, we present a comprehensive guide to strategies that will help you deliver smoother, more responsive, and more efficient apps. Use our tips to optimize your creations and unlock the full potential of Flutter.
Excessive layout nesting
When we talk about excessive layout nesting, we refer to the practice of layering multiple widgets, one inside the other, often leading to a complicated widget tree. While Flutter’s flexible and expressive nature permits you to create intricate layouts, it’s essential to strike a balance between complexity and simplicity.
There are multiple drawbacks to excessive nesting:
- It can lead to decreased performance as Flutter must traverse a deep widget tree during the rendering process, consuming more memory and processing power.
- It makes your code harder to read, understand, and maintain.
- It may result in unintentional bugs and hinder your ability to make quick modifications to the UI.
To mitigate these issues, Flutter developers should employ techniques like using layout widgets in moderation, employing the LayoutBuilder widget when necessary, and keeping a watchful eye on the widget tree’s depth. It’s crucial to prioritize the use of widgets that serve specific layout purposes, such as Column, Row, and Stack while avoiding excessive reliance on generic containers like Container and Padding.
By adhering to a strategy that minimizes excessive layout nesting, developers can create Flutter applications that are not only high-performing but also maintainable and adaptable, ultimately leading to a superior user experience and a streamlined development process.
Image compression
Image compression in Flutter is a crucial optimization technique employed to enhance application performance and minimize resource consumption. It involves reducing the file size of images without compromising their visual quality. By effectively compressing images, Flutter developers can create applications that load faster, occupy less storage space, and conserve network bandwidth.
When implementing image compression in Flutter, developers can choose from a range of strategies, including lossless and lossy compression techniques. Lossless compression retains the image’s original quality while decreasing its size, making it suitable for graphics that require high fidelity. Lossy compression, on the other hand, achieves higher compression ratios by sacrificing some quality, making it more suitable for images where a slight reduction in quality is acceptable.
Flutter provides developers with various packages and plugins, such as the flutter_image_compress package, to facilitate image compression[1]. You can also use external tools to convert images into more efficient formats, such as WebP. These solutions enable you to adjust parameters like image format, quality, and resolution, tailoring the compression to your specific needs.

Source: https://seomelbourne.com/seo-optimisation/webp-image-format-speed-up-website/
Moreover, responsive design techniques can be combined with image compression to load images of different resolutions and sizes based on the device’s screen size and resolution. This further optimizes performance by reducing unnecessary data transfer and rendering of oversized images.
Lazy loading
This strategy helps manage and improve the performance of your applications by deferring the loading of images and data until they are actually needed. This approach is particularly useful when dealing with extensive lists or grids of items, such as those found in news feeds, galleries, or data-heavy applications.
In Flutter, lazy loading of images and data works by loading content on-demand as it comes into the user’s view rather than preloading everything at once. This strategy conserves resources, reduces data usage, and speeds up the initial app loading time. Lazy loading is particularly advantageous when dealing with large datasets or remote resources. By fetching data incrementally, you avoid overwhelming the device’s memory.
A popular example of a mobile application using infinite scroll is Instagram:

Source: https://peppermintcreate.com/what-does-infinite-scroll-mean-for-adword-users/
Minimizing memory usage
To minimize memory usage and prevent memory leaks, developers should focus on proper resource disposal, such as closing streams and controllers when they are no longer needed. Additionally, using Flutter’s static analysis tool and memory profiling tools can help identify and address memory-related issues.
It’s crucial to avoid global variables and singletons that may lead to long-lived objects in memory and to consider using efficient image-loading techniques, stateless widgets, and isolates for heavy computations.
Code splitting
Code splitting is a technique used in Flutter to optimize application performance by dividing the codebase into smaller, more manageable chunks. The goal is to load only the necessary code and assets, reducing the initial load time and memory usage.
A common approach to code splitting in Flutter is to break down the app into modules or features, each containing its own set of code, assets, and dependencies. These modules can be loaded dynamically when needed, allowing you to delay the loading of less frequently used features until they are actually required.
By splitting your codebase into smaller, logical units, you can achieve several benefits. First, it reduces the initial app load time, as only the essential code is loaded upfront. This is particularly advantageous in scenarios where users expect a quick app launch. Second, memory consumption is optimized because unnecessary code and assets are not loaded into memory until requested. Finally, it improves code maintainability by enforcing a clear and modular structure, making it easier to develop and maintain your application.
In Flutter, code splitting is often implemented using the previously mentioned concept of lazy loading. This is particularly useful when dealing with large applications with many screens, widgets, and resources.
Caching and local storage
Caching involves storing frequently accessed data, such as images, API responses, or other resources, in a local cache. It can be in-memory or on-disk and allows your application to quickly retrieve data without the need to fetch it from a remote server repeatedly.
Local storage, on the other hand, refers to the storage of persistent data on the device itself. It is especially useful for retaining user preferences, settings, and app-specific data. Flutter provides the shared_preferences package for straightforward key-value storage and the sqflite package for working with SQLite databases, allowing you to store structured data locally.
Combining caching and local storage can significantly enhance your application’s performance. By caching frequently used data, you reduce the need for frequent network requests, which can be time-consuming and resource-intensive. Local storage enables you to store data that should persist across app sessions, improving the user experience by preserving user preferences and application state.
7. Multithreading
It is a crucial technique in Flutter for optimizing performance and responsiveness in applications. It involves running multiple threads of execution at the same time, allowing tasks to be performed in parallel. While Dart, the language used in Flutter, has built-in support for asynchronous programming, multithreading provides an additional level of control over concurrent operations.
In Flutter, multithreading is often used to perform time-consuming tasks without blocking the user interface (UI). This is critical to maintain the app’s responsiveness. By moving tasks like data fetching, image processing, or complex computations to separate threads, the main UI thread remains free to handle user interactions and maintain a smooth user experience.
Multithreading in Flutter is particularly beneficial for tasks like decoding images, deconstructing complex data, and performing long computations. By running these tasks in separate threads, you ensure that the UI remains responsive and the user experience is smooth, even when dealing with resource-intensive operations.
However, it’s important to manage communication between threads carefully to prevent data race conditions and other concurrency-related issues, ensuring that multithreading enhances your application’s performance and doesn’t introduce new problems.
State management
State management is a fundamental concept in Flutter that revolves around efficiently handling and updating the data and user interface of an application. It’s essential for building responsive and dynamic applications and avoiding unnecessary re-rendering. Flutter offers various state management techniques, allowing developers to choose the one that best fits their project requirements.
At its core, state management involves managing and sharing the application’s state or data between different parts of the application, such as widgets, screens, or modules. The state can be categorized into two main types:
- Local State – concerns data that is specific to a particular widget or a small portion of the application. Widgets manage their local state using the State object, which allows them to update and refresh their own content without affecting other parts of the application. This is often suitable for simple applications or small, self-contained widgets.
- Global State – also known as app-wide state, refers to data that needs to be shared and accessed across multiple widgets, screens, or even the entire application. Managing the global state effectively is essential for complex applications. Flutter offers various management solutions to handle global state, including Provider, Riverpod, BLoC, and GetX.
Performance testing
One primary aspect of performance testing in Flutter is assessing the app’s rendering speed. This involves measuring how quickly the app can render its user interface, taking into account factors like the frame rate (measured in frames per second, or FPS) and the time taken to build and display each frame. Performance testing tools and packages, such as the built-in Flutter DevTools, help developers monitor rendering performance and identify areas that may need improvement.
Another key consideration in performance testing is evaluating the app’s memory usage. Monitoring memory consumption helps identify potential memory leaks and resource inefficiencies. You can use Flutter DevTools and memory profiling to analyze memory allocation and ensure that the app releases resources appropriately, preventing crashes and slowdowns.
Source: https://dart.dev/tools/dart-devtools
Network performance is also crucial in Flutter applications. Performance testing involves examining the app’s behavior when interacting with remote servers or APIs. It assesses response times, data transfer efficiency, and error handling under various network conditions, ensuring that the app provides a seamless experience for users, even in less-than-ideal network environments.
Performance testing further extends to app startup times, navigation speed between screens, and responsiveness to user interactions. It also helps catch and fix any issues early in the development cycle, reducing the risk of performance-related problems in the final product. These aspects are vital for delivering a snappy and engaging user experience.
Animation optimization
One fundamental principle of animation optimization in Flutter is to minimize the use of heavy or excessive animations. Overloading an application with too many such elements can lead to performance issues. It’s important to use animations purposefully and avoid redundant or distracting effects that do not contribute to the user experience.
To optimize animations in Flutter, consider the following:
- Use tween-based animations: They allow you to specify the beginning and ending states of an animation, enabling precise control over the animation’s behavior.
- Custom easing curves: Use them sparingly to achieve specific visual effects, but keep in mind that complex curves can impact performance.
- Minimize the number of animations: Reducing the number of animations (especially complex ones) helps maintain a fluid user interface.
- Frame rate considerations: For smooth animations, it’s generally recommended to target a frame rate of 60 FPS, but for less critical animations, you can use lower frame rates to conserve resources.
- Use GPU-based animations: They allow applications to maintain high frame rates, offload graphic-intensive tasks from the CPU, and implement complex visual effects efficiently.
Minimizing the use of external resources
This approach involves reducing reliance on external services, assets, and dependencies, which can lead to improved performance, reduced loading times, and greater control over your application.
One way to achieve this is by reducing external network requests. Fetching data from external APIs or servers can introduce latency and dependency on external services. By minimizing the number of network requests, you not only speed up data retrieval but also reduce the risk of network-related issues that can disrupt the user experience. Consider caching data locally to further decrease the need for repeated network requests.
When it comes to external assets, such as images or fonts, keeping them to a minimum can optimize your app’s size and loading speed. Utilizing techniques like image compression, vector graphics, and loading assets on-demand (lazy loading) can help manage external assets more efficiently.
Another aspect to consider is the moderate use of third-party libraries and dependencies. While Flutter has a rich ecosystem of packages and plugins, excessive reliance on external dependencies can bloat your app, increase maintenance complexity, and introduce compatibility challenges. It’s essential to evaluate whether the benefits of a particular dependency outweigh the costs and potential risks.
Opting in to new Flutter features
Opting into new Flutter features is an essential aspect of staying up-to-date with the latest enhancements and capabilities offered by the framework. It allows developers to leverage new features, improvements, and APIs that can streamline development and enhance application performance.
Flutter frequently releases updates and new versions with experimental features and improvements. To opt into these new features, developers can start by updating their Flutter SDK to the latest version. This ensures access to the most recent advancements and fixes. An interesting example is Flutter 2.0, which introduced null safety, a feature that helps eliminate a whole class of bugs.
Once updated, developers can selectively enable new features as needed in their Flutter applications. This can be done through feature flags and configuration options. These flags are typically provided by the Flutter team or specific packages and allow developers to experiment with new features while maintaining backward compatibility with older versions of Flutter.

Source: https://developers.googleblog.com/2021/03/announcing-flutter-2.html
Monitoring and error reporting
To effectively monitor your Flutter application, you can use various tools and strategies. These include integrating monitoring services like Firebase Performance Monitoring or Sentry, which provide real-time insights into app performance, network requests, and error tracking. Such services allow you to collect valuable data, monitor the app’s behavior, and identify performance bottlenecks.
In addition to third-party services, Flutter provides built-in tools for performance monitoring, such as Flutter DevTools. This suite of tools offers insights into the app’s rendering performance, memory usage, and network activity, allowing developers to identify and address issues that may impact the user experience.
Error reporting is a critical part of monitoring, as it helps you understand when and why your app encounters issues. Flutter developers can integrate error reporting services like Firebase Crashlytics or Sentry, which capture error logs, exceptions, and stack traces. This data is vital for diagnosing and resolving issues, as it provides detailed information about the context in which errors occur.

Minimizing the use of external plugins
When you minimize the use of external plugins, you get a more concise and independent codebase. This can facilitate the maintenance of your project, as you have fewer external integrations to manage and update.
Furthermore, you gain greater control over the functionality of your application. Relying less on external plugins means that you’re not bound by the limitations or update schedules of those packages. You can tailor your app’s behavior more precisely to your specific requirements.
However, it’s essential to strike a balance between minimizing external plugins and leveraging the rich ecosystem of Flutter packages. Some third-party plugins can significantly speed up development and offer valuable features that would be time-consuming to implement from scratch. So, choose only high-quality and well-optimized plugins for Flutter.
Optimizing a Flutter application for specific platforms
Each platform, such as Android and iOS, has its own unique characteristics and performance considerations. By tailoring your app to the specifics of the target platform, you can enhance the user experience and make the app feel native on each platform.
One of the differences between Android and iOS is that they handle rendering and animations differently. Understanding these nuances and addressing them appropriately is essential to delivering the best performance and user experience on each platform. If you develop an application for both Android and iOS, remember to tailor optimizations to the specific solution.
These are just basic strategies for performance enhancement in Flutter applications. They will help you effectively optimize your creations and unlock their full potential. Apply them to ensure exceptional experiences for your users!